Django框架搭建
Django搭建基本的操作流程,没有基础知识的讲解
一、Anaconda 虚拟环境安装
1. 安装url
Free Download | Anaconda
2. 环境配置 path
一般安装完成之后自动配置,自己看一眼,基本不需要配置。
1 2 3 4 5 6 7
| C:\Anaconda\Scripts
C:\Anaconda\Library\mingw-w64\bin
C:\Anaconda\Library\usr\bin
C:\Anaconda\Library\bin
|

3. 查看已有环境
conda env list
4. 新建环境
conda create -n mypython37 python=3.7
mypython37为环境名字,python=3.7版本号
5. 使用激活or切换
conda activate mypython37
6. 安装包
1 2
| pip install xxx conda install xxx
|
二、PyCharm安装
社区版 PyCharm
三、Django框架配置
1.安装Django
pip install Django

2.创建Django项目
django-admin startproject HelloDjango

3.创建应用
先切换到你的项目目录下
1 2 3
| cd HelloDjango
python manage.py startapp myApp
|

4.使用Pycharm打开项目
新建两个文件夹
static
templates

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| HelloDjango/ # 项目根目录 │ ├── HelloDjango/ # 项目配置目录 │ ├── __init__.py │ ├── settings.py # 项目配置文件 │ ├── urls.py # 项目的URL配置文件 │ └── wsgi.py # WSGI配置文件 │ ├── myapp/ # 应用目录 │ ├── migrations/ # 数据库迁移文件目录 │ ├── __init__.py │ ├── admin.py # 管理后台配置文件 │ ├── apps.py # 应用配置文件 │ ├── models.py # 模型定义文件 │ ├── tests.py # 测试文件 │ └── views.py # 视图函数文件 │ ├── static/ # 存放静态文件(如 CSS、JavaScript、图片等) │ ├── templates/ # 存放模板文件(HTML) │ └── manage.py # Django管理工具
|
5. settings . py 设置
注册之前创建的应用
1 2 3 4 5 6 7
| INSTALLED_APPS = [
······
'myApp’
]
|

1 2 3
| import os #在文件开头添加
'DIRS': [os.path.join(BASE_DIR, 'templates’)]
|
自带,查看自己项目是否有。
STATIC_URL = '/static/'
1 2 3
| LANGUAGE_CODE = 'zh-hans'
TIME_ZONE = 'Asia/Shanghai'
|
6. 启动项目
在pycharm中或者在之前Anaconda Prompt中都可以,前提是要切换到当前的项目目录下。

python manage.py runserver

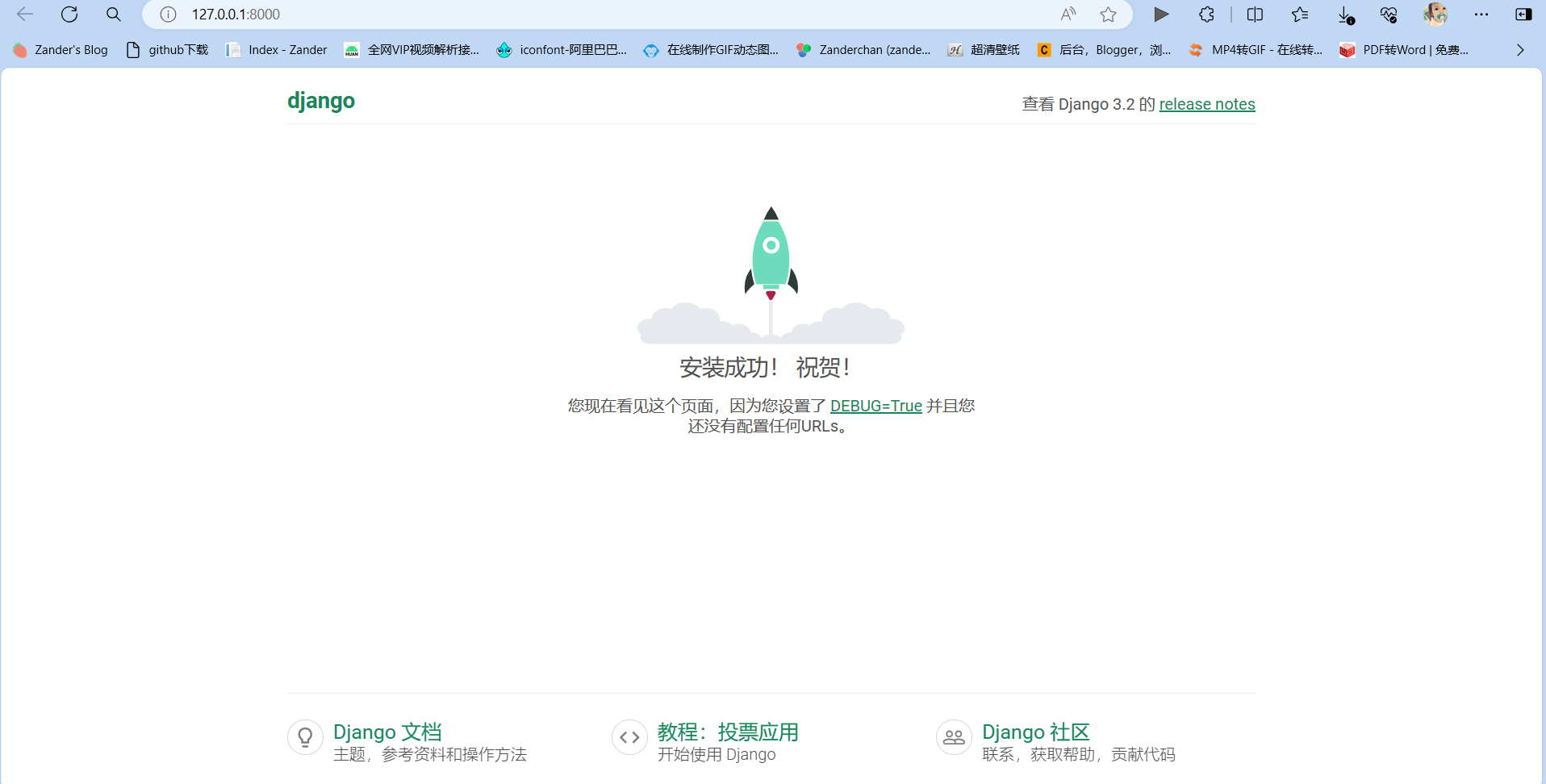
四、MySQL和Navicat 安装
MySQL 下载
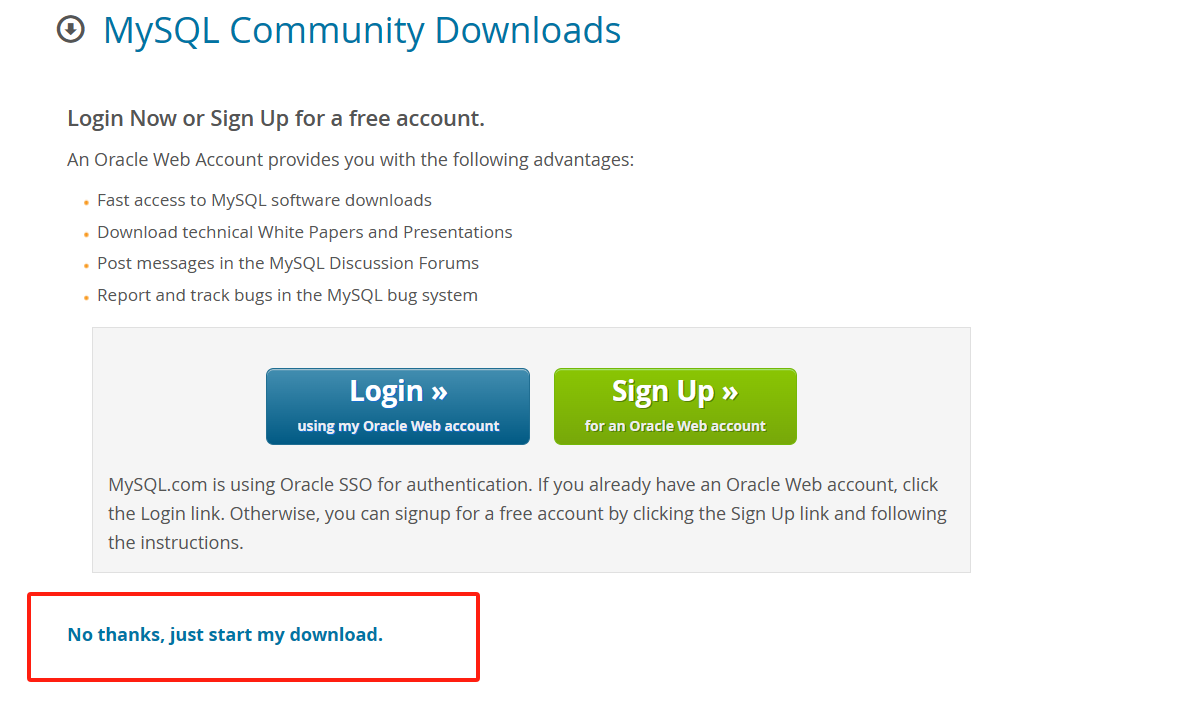
参考博客安装mysql
参考博客激活Navicat Premium15
参考博客激活Navicat Premium16
五、项目配置MySQL
1. 创建数据库
打开Navicat,新建MySQL连接。
用户名密码输入安装MySQL时的用户名密码。
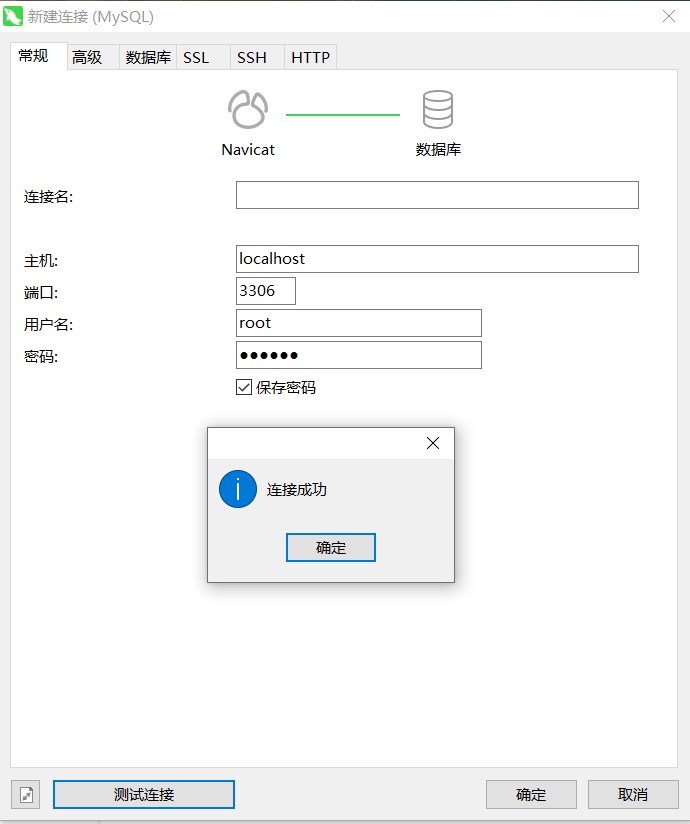
新建数据库,mydb名字自己取
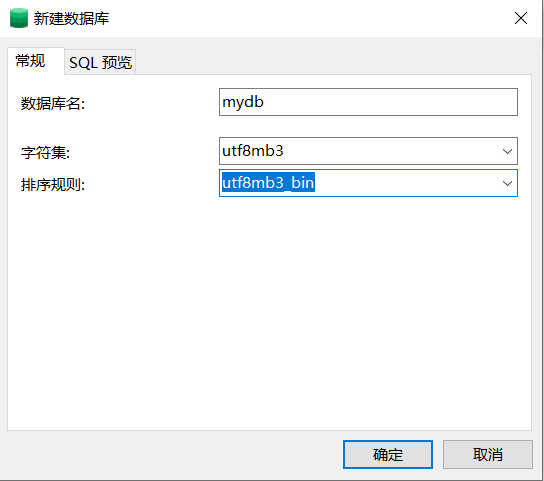
2.在项目中配置Django
settings.py
1 2 3 4 5 6 7 8 9 10
| DATABASES = { 'default': { 'ENGINE': 'django.db.backends.mysql', 'NAME': 'mydb', #你得数据库名字 'HOST': 'localhost', 'PORT': 3306, 'USER': 'root', #你的数据库账号 'PASSWORD': '123456' #密码 } }
|
项目下的__init__.py(注意不是app下的)
1 2
| import pymysql pymysql.install_as_MySQLdb()
|

有红色曲线,鼠标放上,安装pymysql
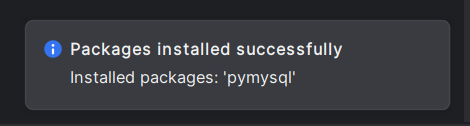
运行项目
1
| python manage.py runserver
|
没有报错,成功!!
3. 实体类 model . py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| class MyUser(models.Model): username = models.CharField(max_length=30, unique=True) password = models.CharField(max_length=128) join_date = models.DateTimeField(auto_now_add=True) phone_number = models.CharField(max_length=11, blank=True, null=True) avatar = models.ImageField(upload_to="avatars/", blank=True, null=True) is_admin = models.BooleanField(default=False)
class Meta: db_table = "user" verbose_name = "用户基础信息" verbose_name_plural = verbose_name
def __str__(self): return self.username
|
python manage.py makemigrations
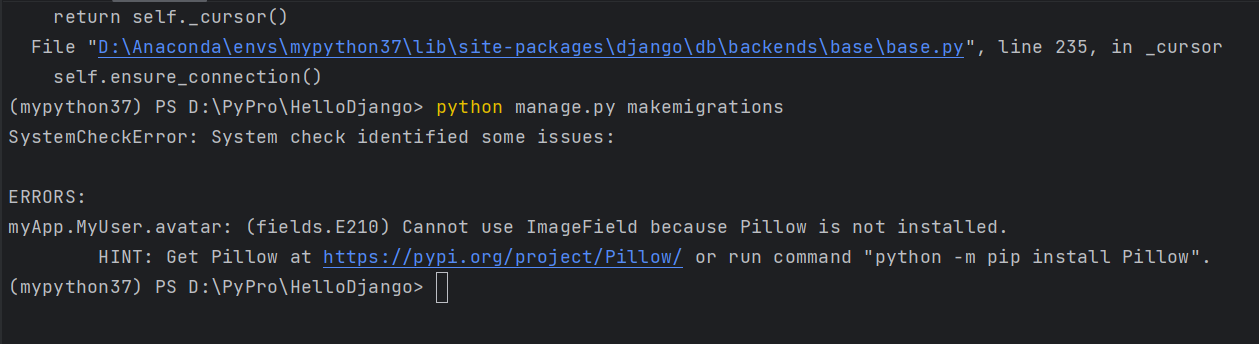
因为上面使用图片ImageField,才会报这个错误,按照他说的错误,安装对应的包。
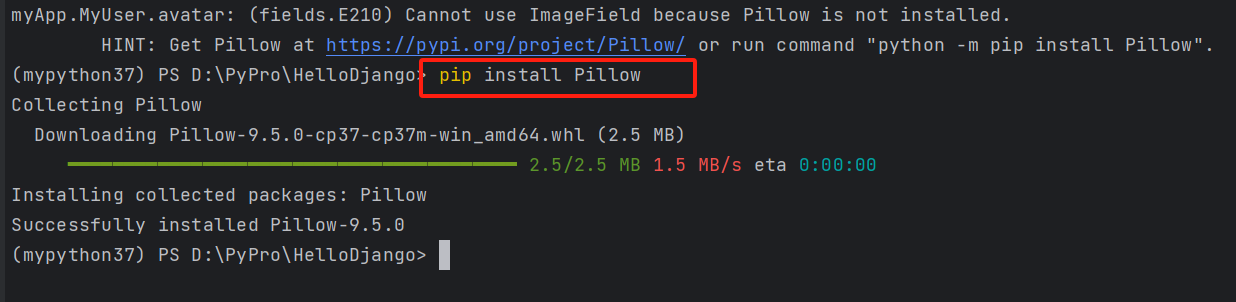
再次执行生成迁移文件

python manage.py migrate
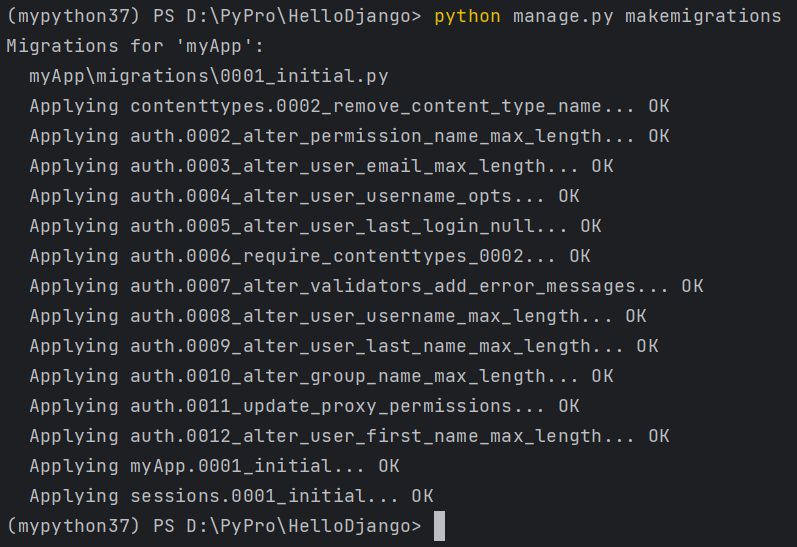
刷新表,出来了一系列的表,其中就有刚刚写的model : User
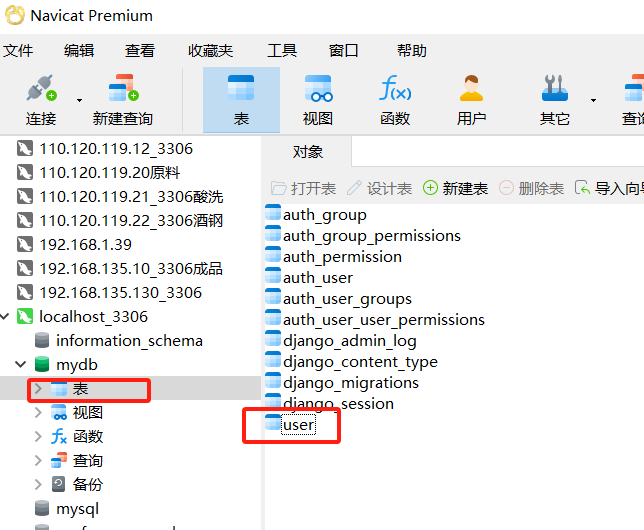
OK!大功告成了!!
六、路由 url. py
1 2 3 4 5 6 7
| from django.contrib import admin from django.urls import path, include urlpatterns = [ path('admin/', admin.site.urls), path("", include("myApp.urls")) ]
|
- 2.复制url. py文件到myapp下
1 2 3 4 5 6
| from django.urls import path from myApp import views urlpatterns = [ path('index', views.index, name='index'), ]
|
七、视图 view. py
可以看到index下有一条黄色的曲线,含义为view.py中没有index这个view(视图),点击创建。
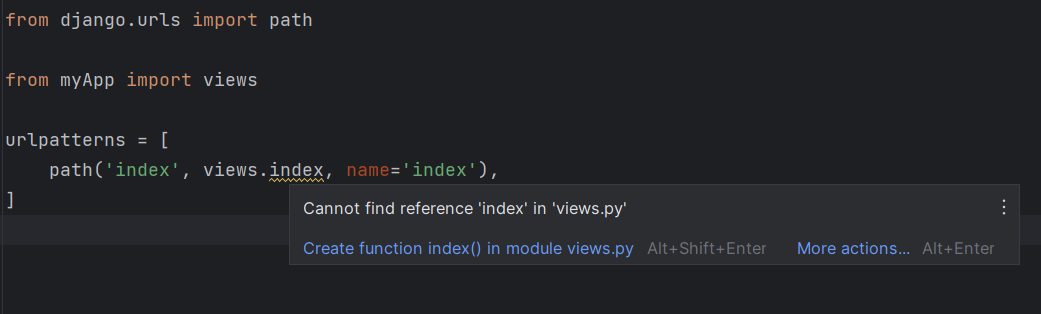
在view.py中创建了一个叫 index()的方法。
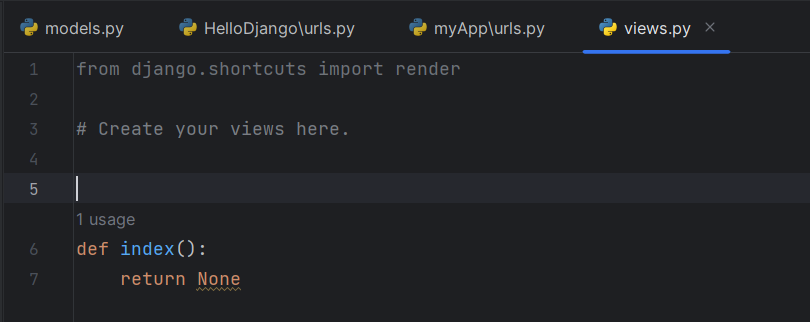
所以说,这个方法名字要和url的名字一一对应。
随便写一个返回看看效果
1 2 3 4
| from django.http import HttpResponse
def index(request): return HttpResponse("Hello world django")
|
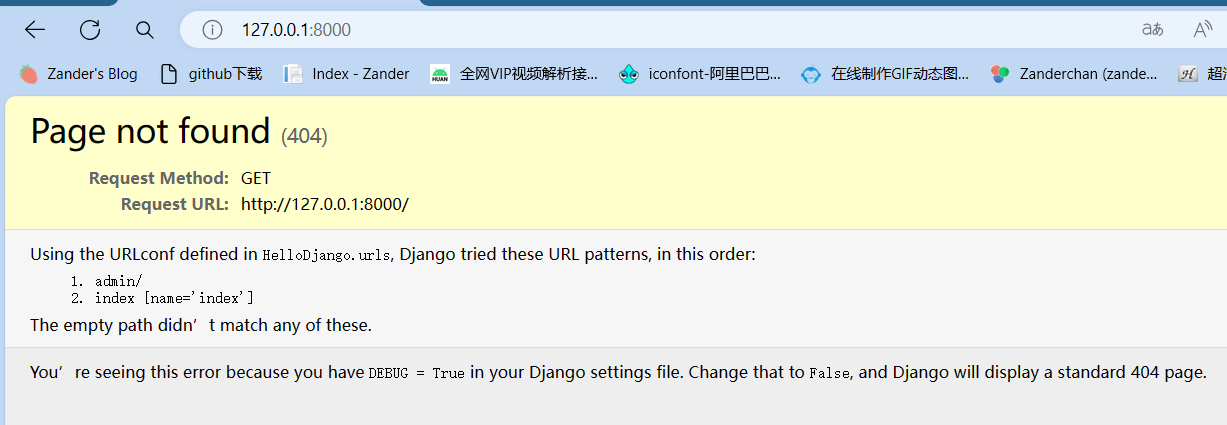
404未正确填写url
url填写正确
127.0.0.1:8000/index
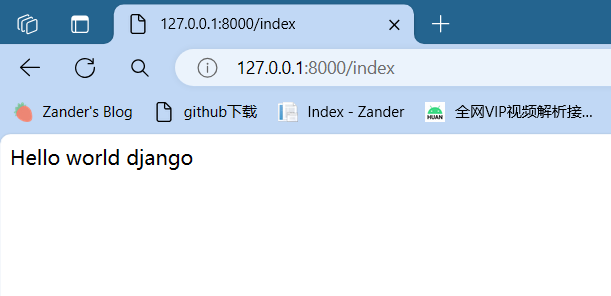
为了方便可以这么写
1 2 3 4
| urlpatterns = [ path('index', views.index, name='index'), path('', views.index, name='index'), ]
|
这样空着也是跳转到index视图。
八、模板文件 templates
模板文件就是我们浏览网站看到的网页html文件。
在模板文件夹下新建一个HTML文件 index.html
前端框架使用 Bootstrap
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.6.2/dist/css/bootstrap.min.css" integrity="sha384-xOolHFLEh07PJGoPkLv1IbcEPTNtaed2xpHsD9ESMhqIYd0nLMwNLD69Npy4HI+N" crossorigin="anonymous"> <script src="https://cdn.jsdelivr.net/npm/jquery@3.5.1/dist/jquery.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script> <script src="https://cdn.jsdelivr.net/npm/bootstrap@4.6.2/dist/js/bootstrap.bundle.min.js" integrity="sha384-7ymO4nGrkm372HoSbq1OY2DP4pEZnMiA+E0F3zPr+JQQtQ82gQ1HPY3QIVtztVua" crossorigin="anonymous"></script> <title>Title</title> </head> <body> </body> </html>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| <body> <table class="table table-hover"> <thead> <tr> <th scope="col">#</th> <th scope="col">First</th> <th scope="col">Last</th> <th scope="col">Handle</th> </tr> </thead> <tbody> <tr> <th scope="row">1</th> <td>Mark</td> <td>Otto</td> <td>@mdo</td> </tr> <tr> <th scope="row">2</th> <td>Jacob</td> <td>Thornton</td> <td>@fat</td> </tr> <tr> <th scope="row">3</th> <td colspan="2">Larry the Bird</td> <td>@twitter</td> </tr> </tbody> </table> </body> </html>
|
1 2
| def index(request): return render(request, "index.html")
|

显示出来的是一个bootstrap格式的表格,即表示bootstrap前端框架使用成功!
九、项目中使用MySQL数据库简单示例
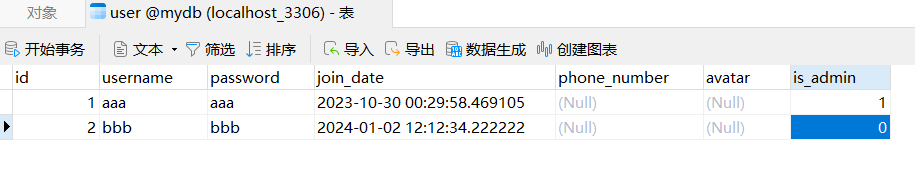
join_date 2023-10-30 00:29:58.469105
手动在这个user表里添加了两条数据,查询这个表的数据,显示在网站上的表格里。
1.查询
view
1 2 3
| def index(request): users = MyUser.objects.all() return render(request, "index.html", {'users': users})
|
index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| <table class="table table-hover"> <thead> <tr> <th scope="col">#</th> <th scope="col">账号</th> <th scope="col">密码</th> <th scope="col">日期</th> </tr> </thead> <tbody> {% for user in users %} <tr> <th scope="row">{{ user.id }}</th> <td>{{user.username}}</td> <td>{{user.password}}</td> <td>{{user.join_date}}</td> </tr> {% endfor %} </tbody> </table>
|
{% %} {{ }}
为Django模板语言
整个操作流程就是这样,有条件的查询参考博客很清晰。
2.修改
1)新建详情界面
修改模板index文件,添加了详情按钮
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| <table class="table table-hover"> <thead> <tr> <th scope="col">序号</th> <th scope="col">账号</th> <th scope="col">密码</th> <th scope="col">日期</th> <th scope="col">操作</th> </tr> </thead> <tbody> {% for user in users %} <tr> <th scope="row">{{ user.id }}</th> <td>{{user.username}}</td> <td>{{user.password}}</td> <td>{{user.join_date}}</td> <td> <a href="/detail?user_id={{ user.id }}"><button type="button" class="btn btn-primary">详情</button></a> </td> </tr> {% endfor %} </tbody> </table>
|
详情按钮加超链接,url后面跟user_id参数
新建detail.html,设置路由,建立详情视图
1 2 3 4 5 6 7
| path('detail', views.detail, name='detail'),
def detail(request): return render(request, "detail.html")
|
detail.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| <!DOCTYPE html> <html lang="cn"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.6.2/dist/css/bootstrap.min.css" integrity="sha384-xOolHFLEh07PJGoPkLv1IbcEPTNtaed2xpHsD9ESMhqIYd0nLMwNLD69Npy4HI+N" crossorigin="anonymous"> <script src="https://cdn.jsdelivr.net/npm/jquery@3.5.1/dist/jquery.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script> <script src="https://cdn.jsdelivr.net/npm/bootstrap@4.6.2/dist/js/bootstrap.bundle.min.js" integrity="sha384-7ymO4nGrkm372HoSbq1OY2DP4pEZnMiA+E0F3zPr+JQQtQ82gQ1HPY3QIVtztVua" crossorigin="anonymous"></script> <title>详情</title> </head> <body> <form> <div class="form-group"> <label for="id" class="sr-only">id</label> <input type="text" readonly class="form-control-plaintext" id="id" name="id" value="{{ user.id }}"> </div> <div class="form-group"> <label for="username">用户名</label> <input type="text" class="form-control" id="username" name="username" value="{{ user.username }}"> </div> <div class="form-group"> <label for="password">密码</label> <input type="password" class="form-control" id="password" name="password" value="{{ user.password }}"> </div> <div class="form-group"> <label for="join_date" class="sr-only">创建时间</label> <input type="text" readonly class="form-control-plaintext" id="join_date" name="join_date" value="{{ user.join_date }}"> </div> <button type="button" class="btn btn-primary">修改</button> <button type="button" class="btn btn-danger">删除</button> </form> </body> </html>
|

2)修改删除功能
修改detail模板文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| <body> <form method="post" action="detail_update"> {% csrf_token %} <div class="form-group"> <label for="id" class="sr-only">id</label> <input type="text" readonly class="form-control-plaintext" id="id" name="id" value="{{ user.id }}"> </div> <div class="form-group"> <label for="username">用户名</label> <input type="text" class="form-control" id="username" name="username" value="{{ user.username }}"> </div> <div class="form-group"> <label for="password">密码</label> <input type="password" class="form-control" id="password" name="password" value="{{ user.password }}"> </div> <div class="form-group"> <label for="join_date" class="sr-only">创建时间</label> <input type="text" readonly class="form-control-plaintext" id="join_date" name="join_date" value="{{ user.join_date }}"> </div> <button type="submit" class="btn btn-primary">修改</button> <a href="detail_del?user_id={{ user.id }}"><button type="button" class="btn btn-danger">删除</button></a> </form> </body>
|
{% csrf_token %}
POST请求需要在表单中包含一个CSRF令牌。
路由url
1 2
| path('detail_update', views.detail_update, name='detail_update'), path('detail_del', views.detail_del, name='detail_del'),
|
视图view
1 2 3 4 5 6 7 8 9
| def detail_update(request): user_id = request.POST.get('id') user = MyUser.objects.get(pk=user_id) username = request.POST.get('username') password = request.POST.get('password') user.username = username user.password = password user.save() return redirect('index')
|
1 2 3 4 5
| def detail_del(request): user_id = request.GET.get('user_id') user = MyUser.objects.get(pk=user_id) user.delete() return redirect('index')
|
3.添加
index.html 加一个按钮
1
| <a href="addUser.html"><button type="button" class="btn btn-success">添加</button></a>
|
创建addUser.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.6.2/dist/css/bootstrap.min.css" integrity="sha384-xOolHFLEh07PJGoPkLv1IbcEPTNtaed2xpHsD9ESMhqIYd0nLMwNLD69Npy4HI+N" crossorigin="anonymous"> <script src="https://cdn.jsdelivr.net/npm/jquery@3.5.1/dist/jquery.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script> <script src="https://cdn.jsdelivr.net/npm/bootstrap@4.6.2/dist/js/bootstrap.bundle.min.js" integrity="sha384-7ymO4nGrkm372HoSbq1OY2DP4pEZnMiA+E0F3zPr+JQQtQ82gQ1HPY3QIVtztVua" crossorigin="anonymous"></script> <title>添加</title> </head> <body> <form method="post" action="add_user_post"> {% csrf_token %} <div class="form-group"> <label for="username">用户名</label> <input type="text" class="form-control" id="username" name="username" required> </div> <div class="form-group"> <label for="password">密码</label> <input type="password" class="form-control" id="password" name="password" required> </div> <button type="submit" class="btn btn-primary">添加</button> </form> </body> </html>
|
路由
1 2
| path('add_user', views.add_user, name='add_user'), path('add_user_post', views.add_user_post, name='add_user_post'),
|
视图
1 2 3 4 5 6 7 8 9
| def add_user(request): return render(request, "addUser.html") def add_user_post(request): username = request.POST.get('username') password = request.POST.get('password') MyUser.objects.create(username=username, password=password) return redirect('index')
|

至此,添加功能完成。
十、完整代码
settings.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120
| import os from pathlib import Path
BASE_DIR = Path(__file__).resolve().parent.parent
SECRET_KEY = 'django-insecure-^ko%qqehu@$)-&i$-4iyq#^nbg3jzz_5!f#(h^99d@c#154nni'
DEBUG = True ALLOWED_HOSTS = []
INSTALLED_APPS = [ 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', 'myApp' ] MIDDLEWARE = [ 'django.middleware.security.SecurityMiddleware', 'django.contrib.sessions.middleware.SessionMiddleware', 'django.middleware.common.CommonMiddleware', 'django.middleware.csrf.CsrfViewMiddleware', 'django.contrib.auth.middleware.AuthenticationMiddleware', 'django.contrib.messages.middleware.MessageMiddleware', 'django.middleware.clickjacking.XFrameOptionsMiddleware', ] ROOT_URLCONF = 'HelloDjango.urls' TEMPLATES = [ { 'BACKEND': 'django.template.backends.django.DjangoTemplates', 'DIRS': [os.path.join(BASE_DIR, 'templates')], 'APP_DIRS': True, 'OPTIONS': { 'context_processors': [ 'django.template.context_processors.debug', 'django.template.context_processors.request', 'django.contrib.auth.context_processors.auth', 'django.contrib.messages.context_processors.messages', ], }, }, ] WSGI_APPLICATION = 'HelloDjango.wsgi.application'
DATABASES = { 'default': { 'ENGINE': 'django.db.backends.mysql', 'NAME': 'mydb', 'HOST': 'localhost', 'PORT': 3306, 'USER': 'root', 'PASSWORD': '123456' } }
AUTH_PASSWORD_VALIDATORS = [ { 'NAME': 'django.contrib.auth.password_validation.UserAttributeSimilarityValidator', }, { 'NAME': 'django.contrib.auth.password_validation.MinimumLengthValidator', }, { 'NAME': 'django.contrib.auth.password_validation.CommonPasswordValidator', }, { 'NAME': 'django.contrib.auth.password_validation.NumericPasswordValidator', }, ]
LANGUAGE_CODE = 'zh-hans' TIME_ZONE = 'Asia/Shanghai' USE_I18N = True USE_L10N = True USE_TZ = True
STATIC_URL = '/static/'
DEFAULT_AUTO_FIELD = 'django.db.models.BigAutoField'
|
HelloProject/url.py
1 2 3 4 5 6 7
| from django.contrib import admin from django.urls import path, include urlpatterns = [ path('admin/', admin.site.urls), path("", include("myApp.urls")) ]
|
myApp/url.py
1 2 3 4 5 6 7 8 9 10 11 12 13
| from django.urls import path from myApp import views urlpatterns = [ path('index', views.index, name='index'), path('', views.index, name='index'), path('detail', views.detail, name='detail'), path('detail_update', views.detail_update, name='detail_update'), path('detail_del', views.detail_del, name='detail_del'), path('add_user', views.add_user, name='add_user'), path('add_user_post', views.add_user_post, name='add_user_post'), ]
|
model.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| from django.db import models
class MyUser(models.Model): username = models.CharField(max_length=30, unique=True) password = models.CharField(max_length=128) join_date = models.DateTimeField(auto_now_add=True) phone_number = models.CharField(max_length=11, blank=True, null=True) avatar = models.ImageField(upload_to="avatars/", blank=True, null=True) is_admin = models.BooleanField(default=False) class Meta: db_table = "user" verbose_name = "用户基础信息" verbose_name_plural = verbose_name def __str__(self): return self.username
|
view.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| from django.shortcuts import render, redirect
from django.http import HttpResponse from myApp.models import MyUser def index(request): users = MyUser.objects.all() return render(request, "index.html", {'users': users}) def detail(request): user_id = request.GET.get('user_id') user = MyUser.objects.get(pk=user_id) return render(request, "detail.html", {'user': user}) def detail_update(request): user_id = request.POST.get('id') user = MyUser.objects.get(pk=user_id) username = request.POST.get('username') password = request.POST.get('password') user.username = username user.password = password user.save() return redirect('index') def detail_del(request): user_id = request.GET.get('user_id') user = MyUser.objects.get(pk=user_id) user.delete() return redirect('index') def add_user(request): return render(request, "addUser.html") def add_user_post(request): username = request.POST.get('username') password = request.POST.get('password') MyUser.objects.create(username=username, password=password) return redirect('index')
|
index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.6.2/dist/css/bootstrap.min.css" integrity="sha384-xOolHFLEh07PJGoPkLv1IbcEPTNtaed2xpHsD9ESMhqIYd0nLMwNLD69Npy4HI+N" crossorigin="anonymous"> <script src="https://cdn.jsdelivr.net/npm/jquery@3.5.1/dist/jquery.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script> <script src="https://cdn.jsdelivr.net/npm/bootstrap@4.6.2/dist/js/bootstrap.bundle.min.js" integrity="sha384-7ymO4nGrkm372HoSbq1OY2DP4pEZnMiA+E0F3zPr+JQQtQ82gQ1HPY3QIVtztVua" crossorigin="anonymous"></script> <title>Title</title> </head> <body> <a href="add_user"><button type="button" class="btn btn-success">添加</button></a> <table class="table table-hover"> <thead> <tr> <th scope="col">序号</th> <th scope="col">账号</th> <th scope="col">密码</th> <th scope="col">日期</th> <th scope="col">操作</th> </tr> </thead> <tbody> {% for user in users %} <tr> <th scope="row">{{ user.id }}</th> <td>{{user.username}}</td> <td>{{user.password}}</td> <td>{{user.join_date}}</td> <td> <a href="/detail?user_id={{ user.id }}"><button type="button" class="btn btn-primary">详情</button></a> </td> </tr> {% endfor %} </tbody> </table> </body> </html>
|
detail.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| <!DOCTYPE html> <html lang="cn"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.6.2/dist/css/bootstrap.min.css" integrity="sha384-xOolHFLEh07PJGoPkLv1IbcEPTNtaed2xpHsD9ESMhqIYd0nLMwNLD69Npy4HI+N" crossorigin="anonymous"> <script src="https://cdn.jsdelivr.net/npm/jquery@3.5.1/dist/jquery.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script> <script src="https://cdn.jsdelivr.net/npm/bootstrap@4.6.2/dist/js/bootstrap.bundle.min.js" integrity="sha384-7ymO4nGrkm372HoSbq1OY2DP4pEZnMiA+E0F3zPr+JQQtQ82gQ1HPY3QIVtztVua" crossorigin="anonymous"></script> <title>详情</title> </head> <body> <form method="post" action="detail_update"> {% csrf_token %} <div class="form-group"> <label for="id" class="sr-only">id</label> <input type="text" readonly class="form-control-plaintext" id="id" name="id" value="{{ user.id }}"> </div> <div class="form-group"> <label for="username">用户名</label> <input type="text" class="form-control" id="username" name="username" value="{{ user.username }}"> </div> <div class="form-group"> <label for="password">密码</label> <input type="password" class="form-control" id="password" name="password" value="{{ user.password }}"> </div> <div class="form-group"> <label for="join_date" class="sr-only">创建时间</label> <input type="text" readonly class="form-control-plaintext" id="join_date" name="join_date" value="{{ user.join_date }}"> </div> <button type="submit" class="btn btn-primary">修改</button> <a href="detail_del?user_id={{ user.id }}"><button type="button" class="btn btn-danger">删除</button></a> </form> </body> </html>
|
addUser.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.6.2/dist/css/bootstrap.min.css" integrity="sha384-xOolHFLEh07PJGoPkLv1IbcEPTNtaed2xpHsD9ESMhqIYd0nLMwNLD69Npy4HI+N" crossorigin="anonymous"> <script src="https://cdn.jsdelivr.net/npm/jquery@3.5.1/dist/jquery.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script> <script src="https://cdn.jsdelivr.net/npm/bootstrap@4.6.2/dist/js/bootstrap.bundle.min.js" integrity="sha384-7ymO4nGrkm372HoSbq1OY2DP4pEZnMiA+E0F3zPr+JQQtQ82gQ1HPY3QIVtztVua" crossorigin="anonymous"></script> <title>添加</title> </head> <body> <form method="post" action="add_user_post"> {% csrf_token %} <div class="form-group"> <label for="username">用户名</label> <input type="text" class="form-control" id="username" name="username" required> </div> <div class="form-group"> <label for="password">密码</label> <input type="password" class="form-control" id="password" name="password" required> </div> <button type="submit" class="btn btn-primary">添加</button> </form> </body> </html>
|